In this post, we will go through the implementation of our first Trigger implementation as part of Real Time Salesforce Project Scenario series.
We should always follow the best practices while writing a trigger. If you are not aware of the best practices of writing a trigger, please read the below post as it is a must to know for every Salesforce Developer.
Also Read:
Let’s get into our first Trigger implementation of Real Time Salesforce Project Scenario series.
Implementation
Real Time Salesforce Project Scenario:
When a new Case is created, Check if there is already a Case for the Account associated with the new Case. If Yes, check if the existing Case has the same Origin as the new Case. If Yes, populate the existing Case as Parent Case on the new Case.
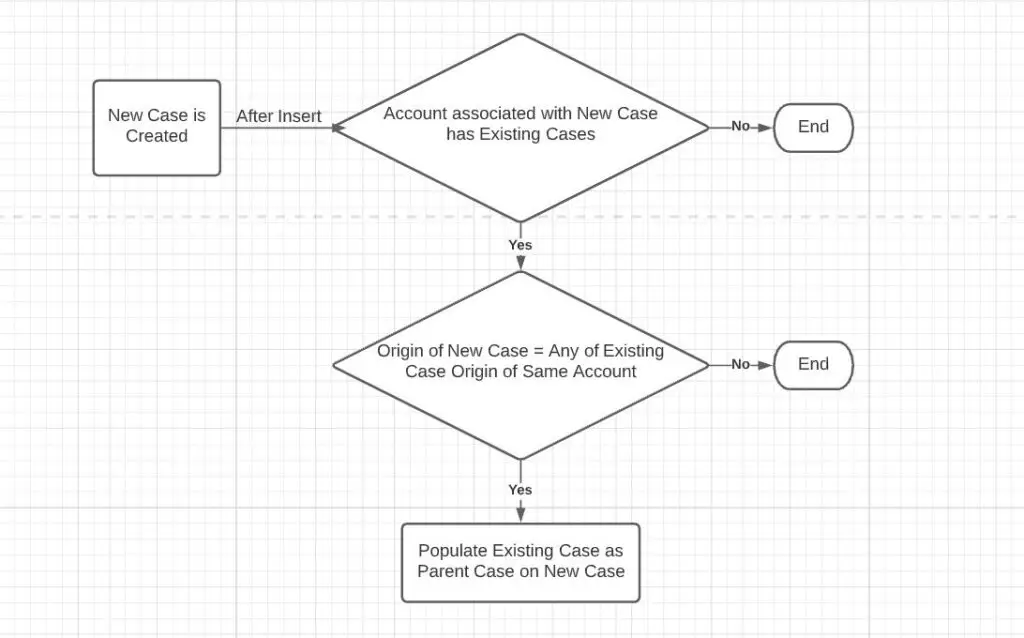
Before writing a trigger, let’s discuss the trigger events.
If we read the scenario carefully, the first thing we notice is “When a new Case is created”. So it should be an insert trigger. But what about after or before the event?
This is tricky. Usually, when we want to create a new record and populate few fields, we can go with the before insert. We can directly update the Trigger.new and we are good. But in this case, we need to write some logic before populating ParentId on the new Case. What if this logic fails and some exception occurs? The new Case will not be inserted in the first place.
Imagine a case is getting created from Email-to-Case. If a customer sends an email to a registered email address, an Case will be created in Salesforce, and the before insert trigger will fire. But if our logic fails and an exception occurs and it is not handled properly, a new Case will not be created.
Also Read:
Hence, in this scenario, it’s better to create an after insert trigger. Because we don’t want to miss out on new Cases in an event of an Exception. But don’t worry, we will cover both after insert and before insert.
Create Case Trigger
Create a Case Trigger with the after insert event. We will keep our business logic in a separate class rather than in a trigger, as it is the best practice to isolate trigger code and business logic. We will just call the method of that Apex class. Our trigger will look something like below:
trigger CaseTrigger on Case (after insert) {
if(Trigger.isAfter){
if(Trigger.isInsert){
CaseTriggerHandler.updateCaseWithParentId(Trigger.New);
}
}
}
Create an Apex Class
This Apex Class will contain the business logic.
Have a look at the below class, then go through the description below it.
public class CaseTriggerHandler {
public static void updateCaseWithParentId(list<Case> newCases){
set<Id> setAccount = new set<Id>();
Map<Id, list<Case>> accountToCase = new Map<Id, list<Case>>();
list<Case> lstCases = new list<Case>();
for(Case c : newCases){
setAccount.add(c.AccountId);
}
list<Account> lstAccount = [SELECT Id, (SELECT Origin, ParentId, AccountId FROM Cases ORDER BY CreatedDate ASC)
FROM Account WHERE Id IN :setAccount];
for(Account a : lstAccount){
accountToCase.put(a.Id, a.Cases);
}
for(Case c : newCases){
for(Case oldCase : accountToCase.get(c.AccountId)){
if((c.Origin == oldCase.Origin) && (c.Id != oldCase.Id)){
lstCases.add(new Case(Id = c.Id, ParentId = oldCase.Id));
break;
}
}
}
update lstCases;
}
}
Real Time Salesforce Project Scenario Logic
- When a new Case is created, we first need to check if its Account has any Cases. So the first thing that we need to do is get the list of all Accounts from new cases that are being created.
- Then we will query the Accounts and it’s associated Cases.
- We need to create Map which will have AccountId as Key and a list of Cases of that Account as Value.
- Then, we need to loop through the new Cases. Add another loop inside new cases to get the list of existing cases for the Account of a current new case.
- Check if the origin of the new Case matched with the Origin of the existing Case. Make sure to add the Id check. Because due to an after insert, the new case is already created in the database and it is also part of existing cases. If both the condition are true, break the loop.
- In the after insert, Trigger.new (newCases) is Read-only. So we cannot directly update the Cases (newCases). We need to create a new instance of Case with Id and then update it.
- In the case of the below insert, we can directly update the Trigger.new (newCases). Please make sure to update the event in the trigger.
That is all from this post, if you don’t want to miss new implementations, please Subscribe here.
I hope you like this new implementation of Real Time Salesforce Project Scenario series.
See you in the next implemetation!
There is no need to use inner for loop. We can create a map to hold the accountId + Case Origin -> Parent Case in second for loop. This map can be used to map the parent case id to the new case in the last for loop.
And since we are updating the same record’s parent id, we can use before insert event. No need to do the explicit DML.
Hi Vamsi, Yes, the code can be optimized a bit but I kept it simple for new learners. Also regarding using before insert event, please go through the post again. I have mentioned why it’s better to use after insert in this scenario.