In this post, we will go through the simple yet important implementation to share records using apex in salesforce. We can manually share a record with a User or a Group. If the owner of the record changes, the sharing is automatically deleted.
For every object in Salesforce, there is a Share object. For Standard objects, the Share object has the below format:
<StanndardObjectAPIName>Share
For example, for Account, the Share object is AccountShare and for Case, it is CaseShare.
For custom objects, the syntax is like below:
<CustomObject>__Share
For example, for custom object Sample__c, the share object would be Sample__share.
Share Record using Apex in Salesforce
In order to share a record using Apex in Salesforce, we need to create a Share object record for the respective record that we want to share. Below are the fields that we need to populate for the share record:
- AccessLevel: The level of access that will be granted to a User or a Group. For standard objects, the name of the field is <ObjectName>AccessLevel like AccountAccessLevel. For custom objects, it is AccessLevel. Available values are Edit, Read, and All. The All access level is an internal value and can’t be used to share records.
- ParentId or StandardObjectId: Id of the record that we want to share. ParentId is only available for Custom Objects. For the Standard objects, use the following format instead of using the ParentId field.
<StandardObject>Id
For Example, to share Account records, use AccountId instead of ParentId for AccountShare. Use CaseId for CaseShare, and so on. - UserOrGroupId: The Id of the User or Group with whom the record will be shared.
- RowCause: This specifies the reason why the record is being shared. manual is the default value.
Below is the sample code to share an Account record with the User. Before going ahead, if you want to know how to share records manually in Lightning, please check this implementation. It also explains to view and edit the share records.
In this implementation, we are going to share an Account record using Apex in Salesforce. Before sharing a record, there is only one Share record for this Account record as you can see in the below screenshot.
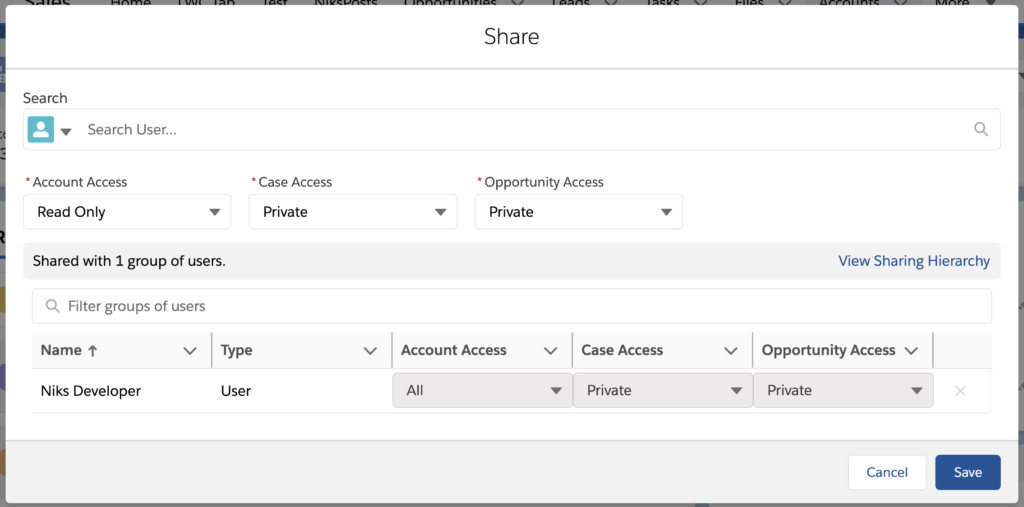
Check the below code to share Account record using Apex in Salesforce:
AccountShare objAccountShare = new AccountShare();
objAccountShare.AccountId = '0012x00000IrBnEAAV';
objAccountShare.UserOrGroupId = '0052x000002Yj03';
objAccountShare.AccountAccessLevel = 'Edit';
// Below 2 Access Levels are mandatory while sharing Account.
// Ignore this for other objects.
objAccountShare.CaseAccessLevel = 'Edit';
objAccountShare.OpportunityAccessLevel = 'Edit';
objAccountShare.RowCause = Schema.AccountShare.RowCause.Manual;
Database.SaveResult sr = Database.insert(objAccountShare,false);
The below Screenshot is the confirmation that the record is shared and now there are 2 Share records.
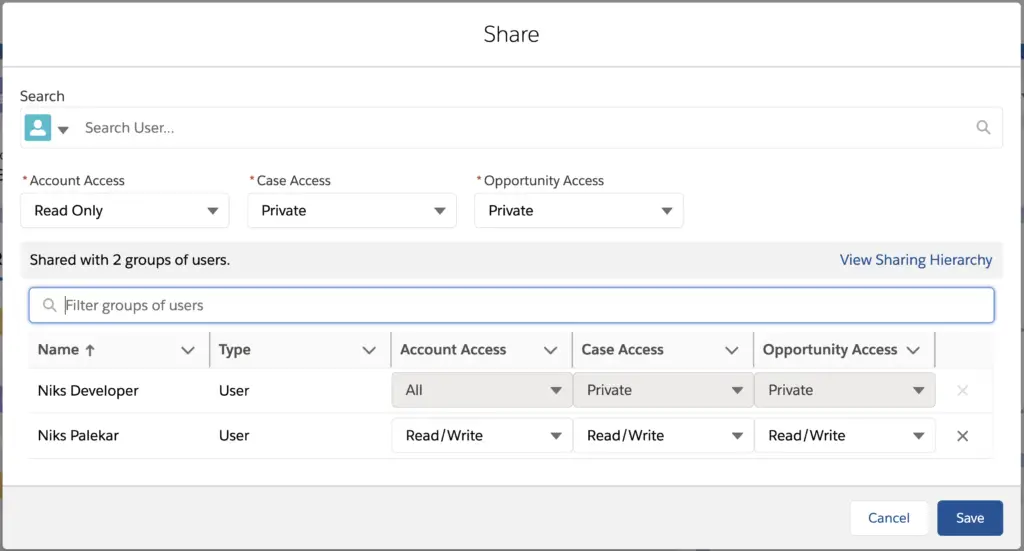
Sample Code for Custom Object
Below is the sample example to share the record of custom object using Apex.
Follower__Share objFollowerShare = new Follower__Share();
objFollowerShare.ParentId = 'a032x00000AViTgAAL';
objFollowerShare.UserOrGroupId = '0052x000002Yj03';
objFollowerShare.AccessLevel = 'Edit';
objFollowerShare.RowCause = Schema.Follower__Share.RowCause.Manual;
Database.SaveResult sr = Database.insert(objFollowerShare,false);
That is all from this post.
If you don’t want to miss new implementations, please Subscribe here.
If you want to know more about how to share records using Apex in Salesforce, check the official documentation here.