In this post, we will implement the functionality to display Toast Notifications in LWC using ShowToastEvent adapter from lightning/platformShowToastEvent module. Using Lightning Web Components, we can display the same type of Toast Notifications as in Lightning Aura Component.
Let’s just get right into the implementation. This is going to be a quick one.
Implementation
We will create four buttons; Success, Information, Warning, and Error to display Toast Notifications.
Create a component toastsComponent and add four buttons with lightning-button-group. Add onclick handler for each button to call the JS Controller method.
toastsComponent.html
<template> <lightning-card title="Display Different Toast Notifications"> <div class="slds-p-left_medium"> <br/> <lightning-button-group> <lightning-button label="Success" onclick={showSuccessNotification}></lightning-button> <lightning-button label="Information" onclick={showInformationNotification}></lightning-button> <lightning-button label="Warning" onclick={showWarningNotification}></lightning-button> <lightning-button label="Error" onclick={showErrorNotification}></lightning-button> </lightning-button-group> </div> </lightning-card> </template>
In JS Controller, add the onchange handler methods for all the buttons. After a button is clicked, the respective method will be called to display the toast.
Display Toast Notifications
To display the Toast Notification in LWC, import ShowToastEvent from lightning/platformShowToastEvent module. Then, create a new instance of ShowToastEvent and add the below parameters:
- title: Title for the Toast Notification
- message: Message to display for the Toast Notification
- variant: To provide the type of Notification. Available values are success, info, warning, and error.
Then, fire the event using this.dispatchEvent(toastInstance) where toastInstance is the ShowToastEvent instance we created earlier.
import { LightningElement } from 'lwc'; import {ShowToastEvent} from 'lightning/platformShowToastEvent'; export default class ToastsComponent extends LightningElement { // To show Success Toast. showSuccessNotification(){ const successToast = new ShowToastEvent({ title : "Success Notification", message : "This is a Success Toast Notification.", variant : 'success' }); this.dispatchEvent(successToast); } // To show Information Toast. showInformationNotification(){ const successToast = new ShowToastEvent({ title : "Information Notification", message : "This is an Information Toast Notification.", variant : 'info' }); this.dispatchEvent(successToast); } // To show Warning Toast. showWarningNotification(){ const successToast = new ShowToastEvent({ title : "Warning Notification", message : "This is a Warning Toast Notification.", variant : 'warning' }); this.dispatchEvent(successToast); } // To show Error Toast. showErrorNotification(){ const successToast = new ShowToastEvent({ title : "Error Notification", message : "This is an Error Toast Notification.", variant : 'error' }); this.dispatchEvent(successToast); } }
This is pretty much it. In addition to the title, message, and variant; there are two more optional parameters that can be provided-
- messageData
- mode
Check official Salesforce documentation here if you want to know more about it.
Display Toast Notifications in LWC
This is how our implementation looks like:
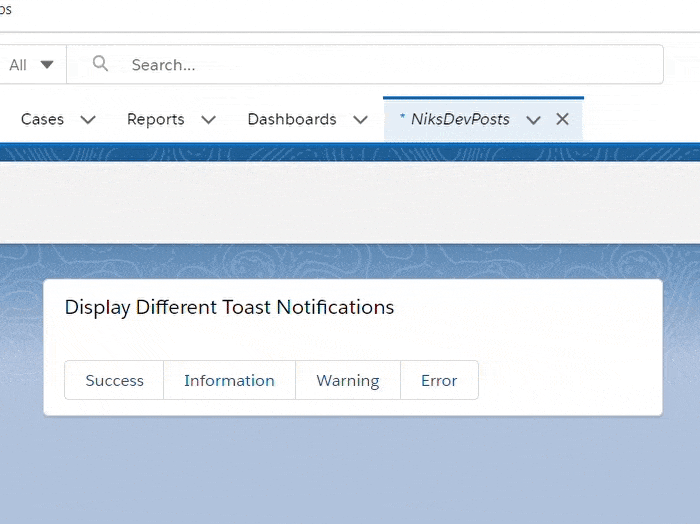
This is how we can Display Toast Notifications in LWC (Lightning Web Components).
Subscribe here if you don’t want to miss new posts. Happy Learning!
If you want to know how to set up Custom Notification for Users, please check this post.
See you in the next implementation, Thank you!