In this post, we will implement different ways to schedule Apex Class in Salesforce. It is very important to schedule Apex Classes in certain business scenarios. This helps to run Apex Classes at specific times, even at regular intervals. Let’s hop into the implementation.
How to make Apex Class schedulable?
To make class schedulable, first, implement the Schedulable Interface. Then you must implement the below method that is defined in Schedulable Interface:
global void execute(SchedulableContext sc){}
The method must be global or public.
Consider the below example, where we have implemented Schedulable Interface and implemented execute method as well. It queries 5 Accounts and updates them.
AccountSchedule.cls
public class AccountSchedule implements Schedulable{
public void execute(SchedulableContext sc){
List<Account> lstAccount = new List<Account>();
for(Account objAccount : [SELECT Type FROM Account WHERE Type != 'Customer - Direct' ORDER BY CreatedDate LIMIT 5]){
objAccount.Type = 'Customer - Direct';
lstAccount.add(objAccount);
}
update lstAccount;
}
}
Schedule Apex Class Declaratively
Now that we have implemented Schedulable Interface, we need to actually schedule an Apex Class, To do that, follow below steps:
- Go to Apex Classes from the Quick Find box.
- Click on Schedulable Apex.
- Select Apex Class that implemented Schedulable Interface and configure the Schedulable Apex Execution, Frequency, Start Date, End Date, and Preferred Start Time. Please make note that we cannot provide Minutes and Seconds for scheduling. It will only run at 0 Minutes and 0 Seconds for specific hour.
This is how the Schedule Apex configuration would look to run Apex Class every day at 12.00 AM for the next 5 years.
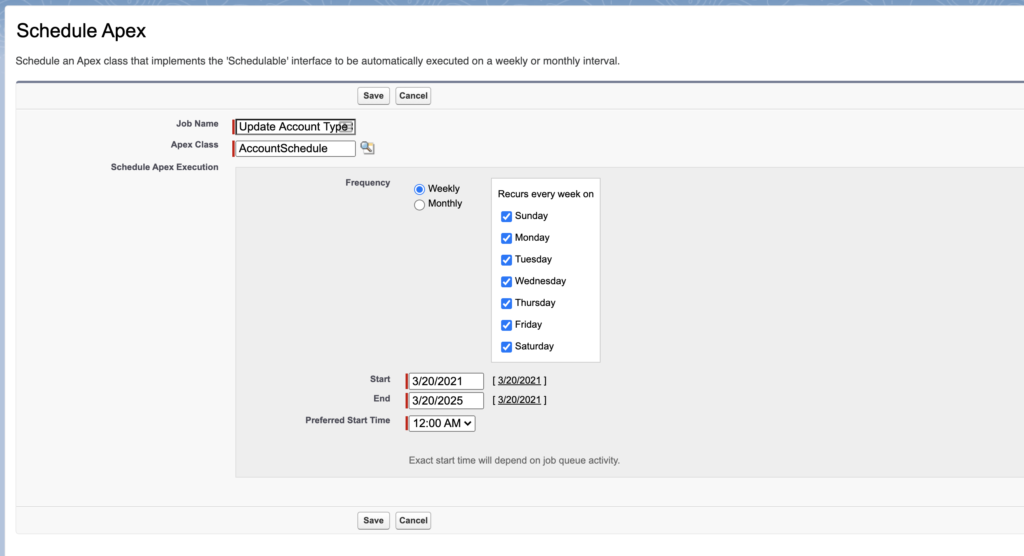
And this is how the Scheduled Job would look in the All Scheduled Jobs:
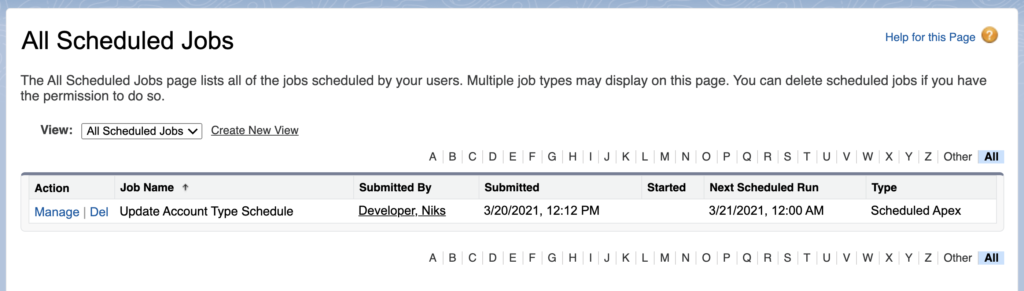
Schedule Apex Class using System.schedule()
We can also schedule an Apex Class using System.schedule(). We need to pass 3 parameters:
- Name of Schedule Job: Any Text value to identify the name for this Schedule Job.
- Expression: An expression used to represent the time and date the job is scheduled to run. Check official documentation to know more about this expression and its format here.
- An instance of an Apex Class that implements Schedulable Interface.
This is how we can call System.schedule() to run Apex Class once a day at 12.00 AM:
System.schedule('Update Account Type Schedule', '0 0 0 * * ?', new AccountSchedule());
Also Read:
Schedule Batch Apex using System.scheduleBatch()
We can use System.scheduleBatch() method to schedule a batch job to run once at a future time. Following are the parameters for System.scheduleBatch():
- An instance of an Apex Class that implements the Database.Batchable interface.
- Name of Schedule Job: Any Text value to identify the name for this Schedule Job.
- The time interval in Minutes after which the job starts executing.
- An optional scope value. By default, it’s 200. Maximum 2000.
Below is the sample Batch Apex that updates 5 Accounts from the database and updates it.
AccountBatch.cls
public class AccountBatch implements Database.Batchable<sObject>{
public Database.QueryLocator start(Database.BatchableContext bc){
return Database.getQueryLocator('SELECT Type FROM Account WHERE Type != \'Customer - Direct\' ORDER BY CreatedDate LIMIT 5');
}
public void execute(Database.BatchableContext bc, List<Account> lstAccount){
for(Account objAccount : lstAccount){
objAccount.Type = 'Customer - Direct';
}
update lstAccount;
}
public void finish(Database.BatchableContext bc){
}
}
And this is how we can schedule this Batch to run after 50 Minutes:
System.scheduleBatch(new AccountBatch(), 'Update Account Type Batch', 50);
These are the different ways to schedule Apex Class in Salesforce.
If you don’t want to miss new implementations, please Subscribe here. If you want to know more about Scheduling Apex Class in Salesforce, please check official Salesforce documentation here.
Thank you! See you in the next implementation!
Thanks a lot for this info Niki.
I have a question, where goes the code (System.schedule()), in which part of the class?
I don’t understand, please send help.
This code:
System.schedule(‘Update Account Type Schedule’, ‘0 0 0 * * ?’, new AccountSchedule());
Hi Karen, you can call it from the Anonymous Window of Developer Console.